Kickstart a Project Using HTML, CSS, and JavaScript
#HTML
#CSS
#JAVASCRIPT
1 min read
|10 months ago
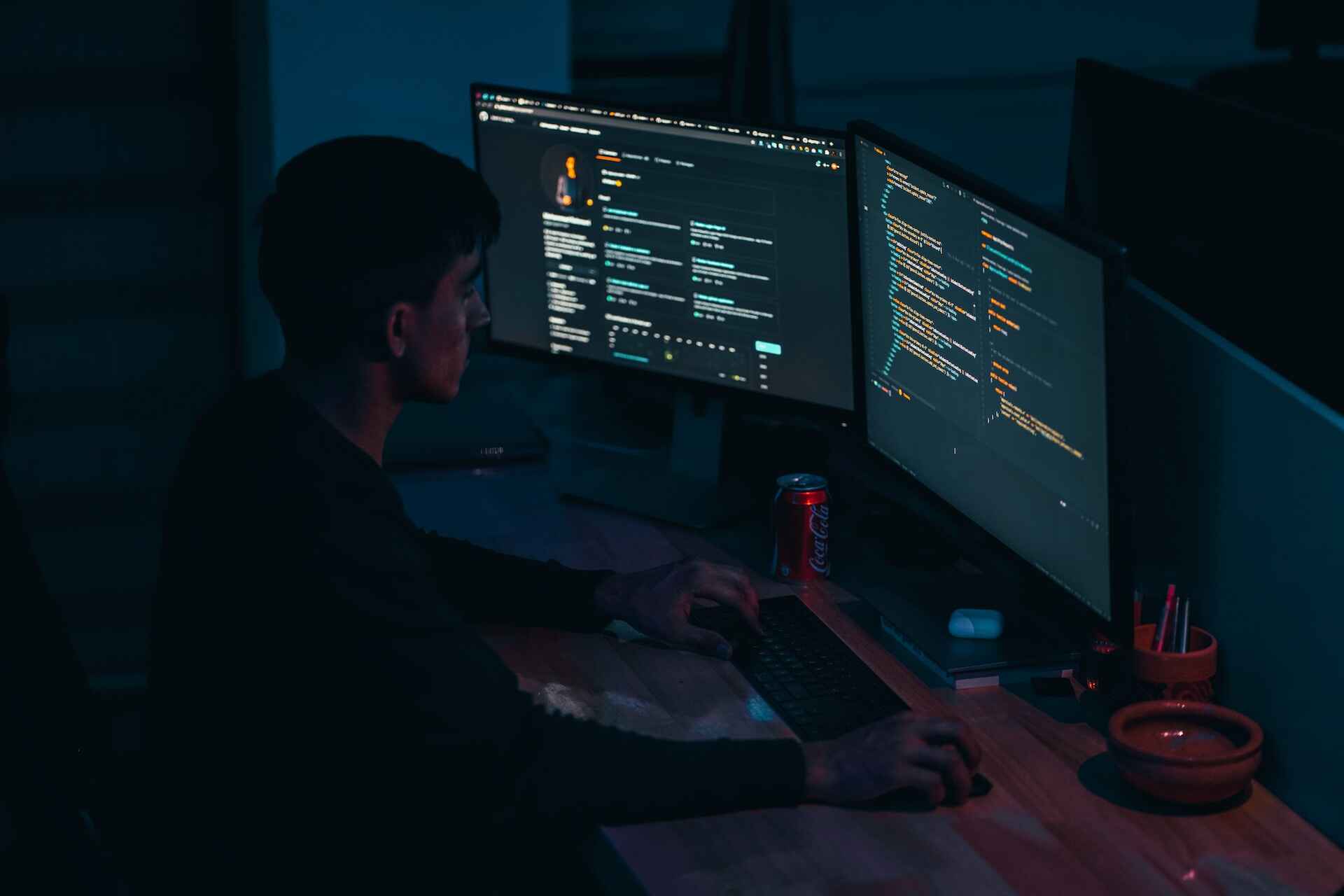
Photo by Mohammad Rahmani on Unsplash
Creating a basic web project with HTML, CSS, and JavaScript is a fundamental skill for any web developer. Here's a step-by-step guide with code examples:
Step 1: Create a Project Folder
Start by creating a new project folder on your computer. You can name it whatever you like. For example, let's call it "my-web-project."
Step 2: Create HTML File
Inside your project folder, create an HTML file (e.g., `index.html`). This will be the main entry point of your website. Here's a minimal HTML structure:
<!DOCTYPE html>
<html>
<head>
<title>My Web Project</title>
<link rel="stylesheet" type="text/css" href="styles.css" />
</head>
<body>
<h1>Hello, World!</h1>
<script src="script.js"></script>
</body>
</html>
Step 3: Create CSS File
Inside your project folder, create an CSS file (e.g., `style.css`) in the same folder. This file will be used to style your HTML content. Here's a simple example:
/* styles.css */
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
}
h1 {
color: #333;
}
Step 4: Create JavaScript File
create a JavaScript file (e.g., `script.js`) in the project folder. This file will contain your JavaScript code. For now, let's add a basic script:
// script.js
document.addEventListener("DOMContentLoaded", function () {
var heading = document.querySelector("h1");
heading.textContent = "Hello, Web Developer!";
});
Step 5: Test Your Project
Open the index.html file in a web browser. You should see "Hello, Web Developer!" displayed with the specified styles from styles.css. The JavaScript code will modify the heading text when the DOM is ready.
Step 6: Optional-Version Control
Consider using a version control system like Git to track changes in your project. Initialize a Git repository in your project folder:
git init
Step 7: Start developing
You're all set! You now have a basic HTML, CSS, and JavaScript project ready. You can start adding more HTML content, styles, and JavaScript functionality to build your web project further.
Remember to keep your project organized and use best practices as you develop and expand your web application.
Loading...